Xamarin 4 has everything you need to create great mobile apps!
Let’s take a look into some of the features and enhancements included in Xamarin 4.0 for developing cross-platform applications including Android, iOS and UWP (Universal Windows Apps).
Introducing Xamarin Forms 2.0
This time I made a simple app called “Lucky Winner” for randomly select a person from a list of participants using the commonly used System.Random class for random numbers just like any other .Net program.
Here are some screenshots of the app running on different platforms:
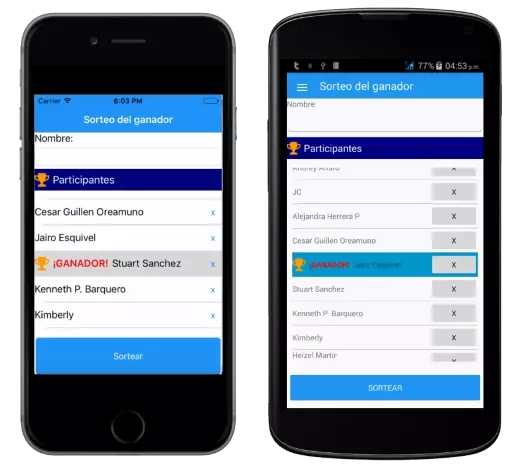
You can download the full source for this app from my GitHub repository here.
Developing a Xamarin.Forms app from scratch
By installing Xamarin Platform and geting a valid/trial license you will be able to create a PCL (Portable Class Library) that contains most of the code for you to be shared and compile native apps for Android, iOS and UWP.
So let’s pick the Xamarin.Forms template from the available list inside Visual Studio:
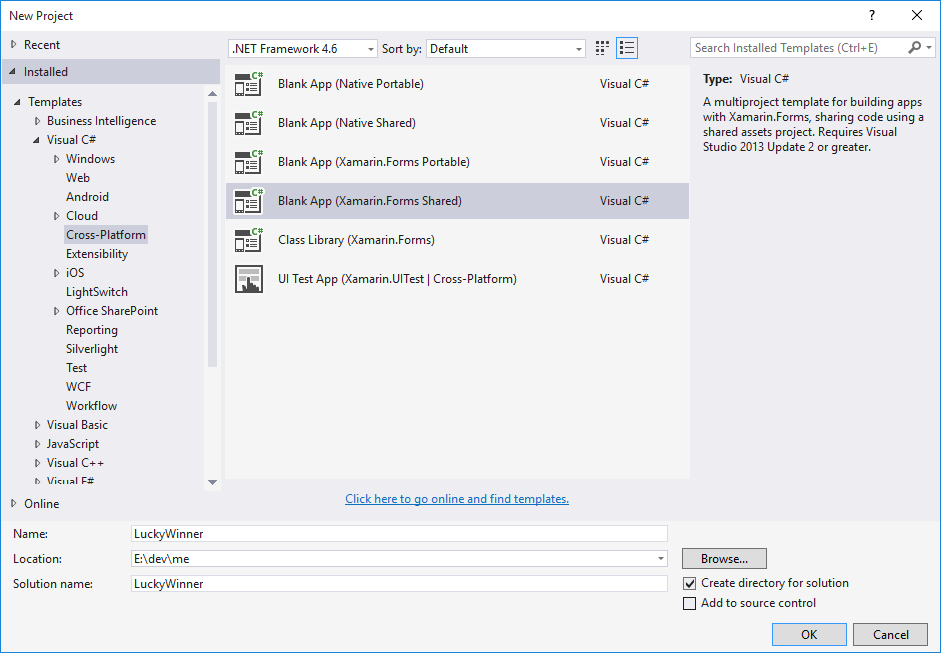
By doing so will get:
- A Xamarin.Forms project for sharing XAML/C# code between platforms
- Android project referencing Xamarin.Forms
- iOS project
- UWP project
If you are familiar with MVVM, XAML and C# it will be quite familiar to easily include Pages, ViewModels, Views in your project just like the following.
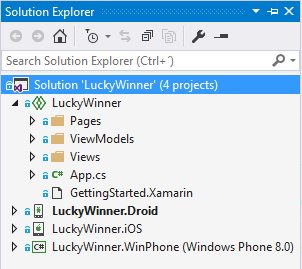
Building User Interfaces with MVVM, in a cross-platform way
Let’s take a look at the XAML code used for defining the list of participants:
...
<ListView x:Name="PlayersSelector"
ItemsSource="{Binding Path=Players}"
SeparatorColor="Gray"
SelectedItem="{Binding Path=Winner, Mode=TwoWay}">
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<ViewCell.ContextActions>
<ToolbarItem Command="{Binding DeleteCommand}" Text="Borrar" />
</ViewCell.ContextActions>
<Grid>
<StackLayout Orientation="Horizontal"
HorizontalOptions="Start">
<Image IsVisible="{Binding Path=IsWinner}"
Source="Prize.png"
HeightRequest="25" WidthRequest="25" />
<Label Text="¡GANADOR!"
IsVisible="{Binding Path=IsWinner}"
TextColor="Red"
FontAttributes="Bold"
VerticalTextAlignment="Center"
/>
<Label Text="{Binding Path=PlayerName}"
VerticalTextAlignment="Center"
/>
</StackLayout>
<Button HorizontalOptions="End"
Command="{Binding Path=DeleteCommand}"
Text="x" />
</Grid>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
The Binding element acts like the glue between your View and ViewModels for allowing you to have clear separation of concerns between them. Other common features supported for Xamarin.Forms with MVVM include Two-way binding, Command Pattern and Value Converters.
Getting a lucky winner with C# just like in any other program.
Remember how to generate a random number in C# but not sure how do get it done for a mobile app? No worries! You can use standard System core features in C# (even 6.0 features) plus other cool features including lamda expressions, delegates, actions, Linq and async operations.
private void Play()
{
...
var random = new Random(DateTime.Now.Millisecond);
var lucky = random.Next(0, ViewModel.Players.Count);
var selectedPlayer = ViewModel.Players.ElementAtOrDefault(lucky);
if (selectedPlayer != null)
{
selectedPlayer.IsWinner = true;
ViewModel.Winner = selectedPlayer;
PlayersSelector.ScrollTo(selectedPlayer, ScrollToPosition.MakeVisible, true);
}
}
Bring Material Design to your Android application
Another great feature for Xamarin for Android is ability to easily incorporate Material Design into your Android apps. This can be done by adding the Support Design Library available as a package from NuGet.
Additional resources & links.
Introducing Xamarin 4 Adding Material Design to Xamarin Apps Lucky Winner source code