Getting your next mobile app connected with Azure Mobile Apps
New to Azure? Need a backend for your mobile app? Here here are key considerations and tips for start developing mobile apps with Azure Mobile Apps.
In a world of mobile-connected devices, one of the primary components for your app is a backend, which provides security, availability and scalability according to your requirements, audience and/or storage capabilities. Instead of doing everything on the user’s device, your app might choose to rely certain responsabilities like complex business processes and time-consuming tasks without compromising the user security and device’s memory.
Meet Azure Mobile Apps:
Azure Mobile Apps is a set of cloud services that allows you to quickly build and run mobile backends with a set of RESTful and OData services through a set of SDKs for connecting programming different stacks including JavaScript, C#, Objective-C, Swift, Java, Cordova and more, which provides a wide-open development board across web, hybrid and native platforms.
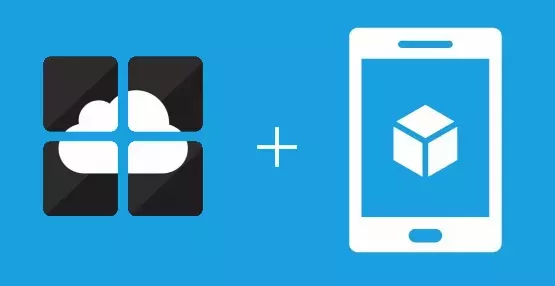
This is extremely important for organizations moving from on-premises environments to cloud services and new app opportunities that require highly-available cloud solutions and common mobile features including:
- Push notifications
- Offline-availability
- Social integrations
- Backend-offline sync
- Auth.
- And more.
Connection awareness and offline sync.
Handling device-disconnected situations is an important feature to prevent your apps to stop working and be useless for your users when your device is offline. Even better, resilent services allow applications to be aware of losing connectivity and sync with your backend when connection is back. There are some cloud products that support this feature including CouchBase’s Mobile, Google’s Firebase, Amazon’s Cognito and Azure as well.
With Azure Mobile Apps, you can take advantage of offline and sync features by having:
- End-to-end JavaScript/.Net based systems.
- Highly-extensible backend with more recent Asp.Net Core / NodeJS features.
- Corporate Sign-On and Active-Directory integrations.
- On-premises extensibility into the cloud.
- And more.
Note: Azure Mobile Apps was previously known as Azure Mobile Services, which has been renamed these days for transitioning to Azure App Service. If you have already been using Azure Mobile Services we recommend you to take look on the transition notes here.
Getting started with Mobile and Azure.
In a nutshell, with Azure Mobile Apps you can create mobile backends in two ways:
- Easy Tables (No-code APIs)
- Full Azure Mobile Apps Backends (NodeJS/.Net)
Here the steps for start using Easy Tables and easily set up a mobile backend in minutes:
1. Setup the Mobile App from Azure portal.
From Azure Portal you can go to New -> Web + Mobile -> Mobile App to start creating your mobile backend and choose the public Azure URL for it:
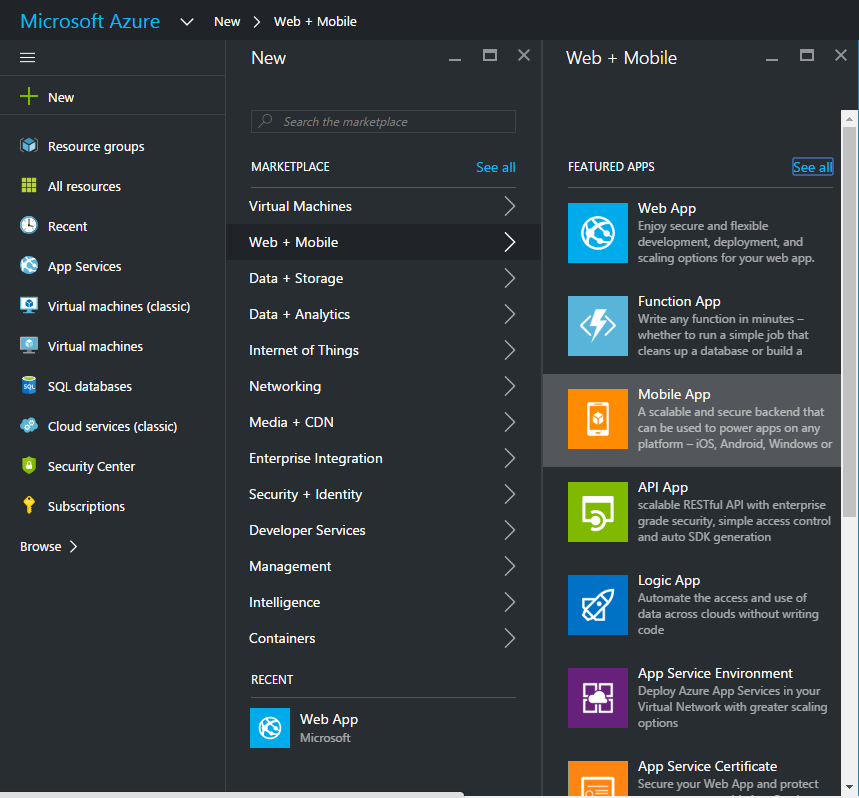
2. Define your Easy Tables schema.
From the Azure portal you can setup your table schema quickly and define the fields that you need to store:

By default, the No-code APIs relies on its own in-built DB, which you can eventually swap into any other SQL/NoSQL persistance of your choice supported by Azure.
3. Start using the REST endpoint.
You can bring your favorite REST debugging tool for testing your no-code API and start using the GET, PUT, UPDATE and DELETE verbs over it.
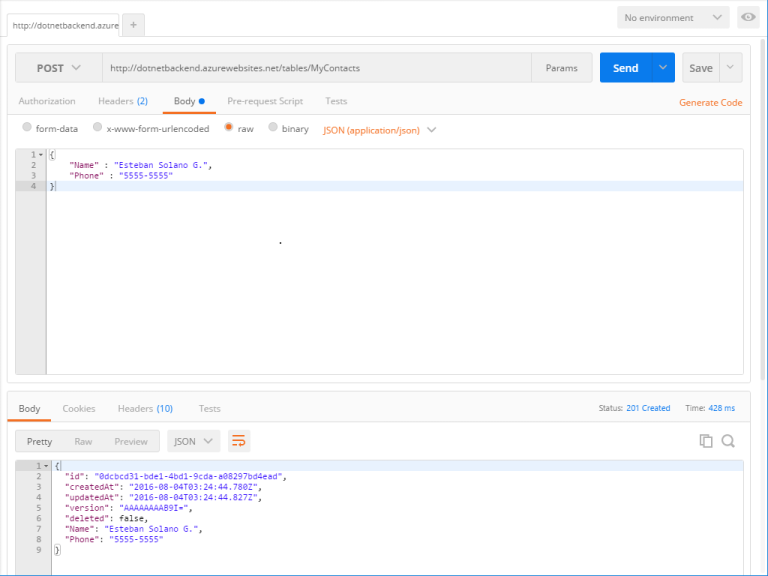
Make sure to include the ZUMO-API-VERSION header with the proper version (2.0.0) to the REST debugging tool of your choice (which is Postman in this case).
Tips for connecting it to your mobile app
- Bring the proper SDK for your platform (Swift, Java, Cordova, Xamarin)
- Configure your client instance (URL) in your app and map your DTOs (Data-Transfer Objects)
- Start using it
- Bring the SDK for your mobile app.
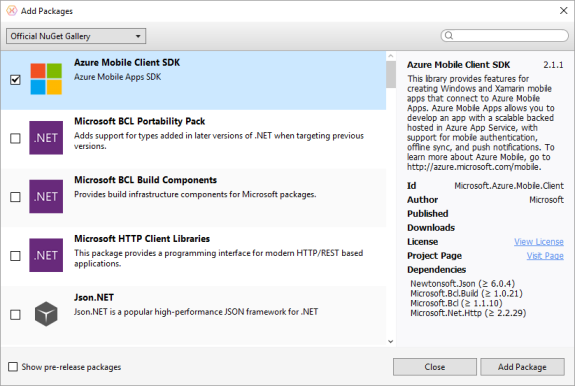
- Configuring your app and DTOs
namespace YourNextMobileApp
{
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
public class Contact
{
[JsonProperty("Id")]
public string Id { get; set; }
[JsonProperty("Name")]
public string Name { get; set; }
[JsonProperty("Phone")]
public string Phone { get; set; }
[Version]
public string Version { get; set; }
}
}
- Tip: Abstract Mobile Mobile Apps with your own ICloudService to be used through your app.
public interface ICloudService
{
Repository<Contact> Contacts { get; }
void Initialize();
}
public class AzureCloudService : ICloudService
{
private IMobileServiceClient _client;
public Repository<Contact> Contacts { get; set; }
public void Initialize()
{
_client = new MobileServiceClient(APP.KEYS.BACKEND_URL);
Contacts = new Repository<Contact>(_client);
}
}
By abstracting your Azure implementation it gets easier for mocking and keep dependencies down through your app.
- Then the Repository implementation
namespace YourNextMobileApp
{
using Microsoft.WindowsAzure.MobileServices;
using System.Collections.Generic;
using System.Threading.Tasks;
using System;
public class Repository<T>
where T : class
{
IMobileServiceClient _proxy;
protected IMobileServiceTable<T> Table { get; private set; }
protected Logger Logger { get; set; }
public Repository(IMobileServiceClient proxy)
{
_proxy = proxy;
Table = _proxy.GetTable<T>();
Logger = new Logger();
}
public async Task<IEnumerable<T>> Get()
{
var empty = new T[0];
try
{
return await Table.ToEnumerableAsync();
}
catch (Exception ex)
{
Logger.Trace(ex);
return empty;
}
}
}
}
Additional Repository instances can be added layer and keep it organized.
- Tip: Keeping your mobile app responsive with async calls
private async void FetchAsync()
{
var result = await Repository.Get();
if (result.Any())
{
foreach (Contact singleItem in result)
{
_observable.Add(new ContactViewModel (singleItem));
}
}
}
- Demo: Fetching the data (Android / Google Maps)
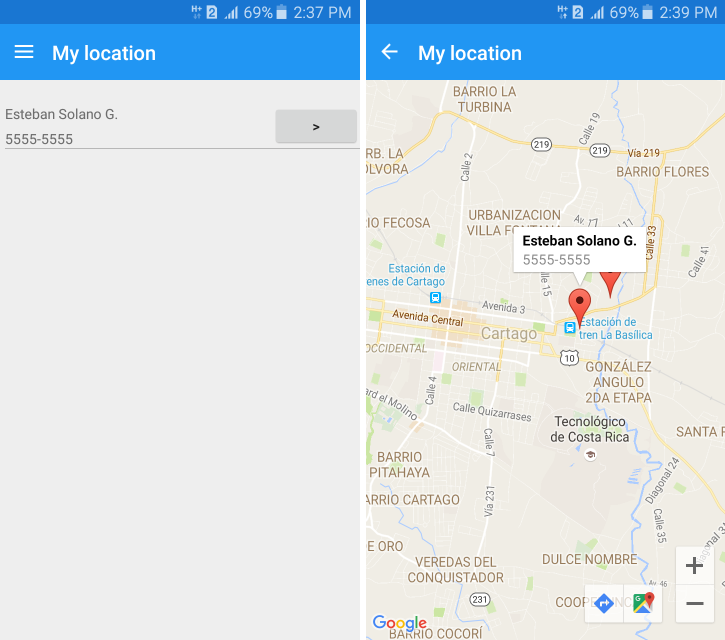
Easy Tables vrs. Azure Mobile Apps - Which one to choose?
While Azure Easy Tables are quick and powerful, the underlying backend relies on a NodeJS implementation, which might not worry you about at least you want to extend it with JavaScript. Alternatively, you can provide your own C#-based Mobile backend on existing Azure SDK tooling and extend your API implementation as needed.
Here are some key considerations for choosing between Azure Easy Tables and Azure Mobile Apps with a .Net/NodeJS backend:
+ Pros of Easy Tables:
- Rely on Azure Cloud with minimum configuration.
- Quick backends deployed in minutes.
- Quickly jump into the SDK for getting more features with your favorite programming language.
- Recommended for App-first scenarios with minimum backend dependencies.
- Cons of Easy Tables:
- Limited custom-logic and customizations.
- Might make your app heavy with too much client-side processing.
- Object-relationships cannot and should not be handled in Easy Tables.
- Extra-operations and custom business logic will need additional tweaks on NodeJS backend (JavaScript).
In the other side, a fully Azure Mobile App backend allows you to bring additional logic layers that be extended with your own backend code with NodeJS / Asp.Net Core and host it on Azure.
+ Pros of Azure mobile-backend implementations:
- Built your own service with Azure flavored features.
- Supporting latest Asp.Net Core / NodeJS features.
- Integration services and extensibility in the Cloud
- Cons of Azure mobile-backend implementations:
- SDKs still being developed (be aware of breaking changes)
- Requires deeper understanding of Azure building-blocks.
Wrapping up.
So far, we explored some of the basics of bringing Azure Mobile Services into your next mobile app. As we saw, you can start with Easy Tables in minutes as a way of setting up a mobile backend right out the door directly from the Azure Portal and start using the REST/OData endpoints directly from your app.
Also, we examined some of the scenarios when you might need or not your own coded backend, and some key considerations before transitioning from Easy Tables to a full Azure Mobile App backend in case you need it.
By using one or the other, you can take the Azure Mobile SDKs available to your development stack and start right the way with a lot features out-the-box for mobile apps including offline-sync and social integrations.
Hopefully those tips can help you to take important features from your Azure box and help you to try or decide to use Azure for your next mobile app or not.
Happy coding!
Recommended resources:
Xamarin - Mobile apps with .Net and Azure
- Azure, Microsoft solution for Cloud Computing
- Xamarin 4 has everything you need to create great mobile apps!
- Get started with Xamarin Test Cloud
- Getting started with Azure Easy Tables
- Add a backend to your app in 10 minutes
- Azure + Xamarin documentation